An effective and useful way to find out whether we are connected to internet or not. Let's show the user's network connectivity in the App.
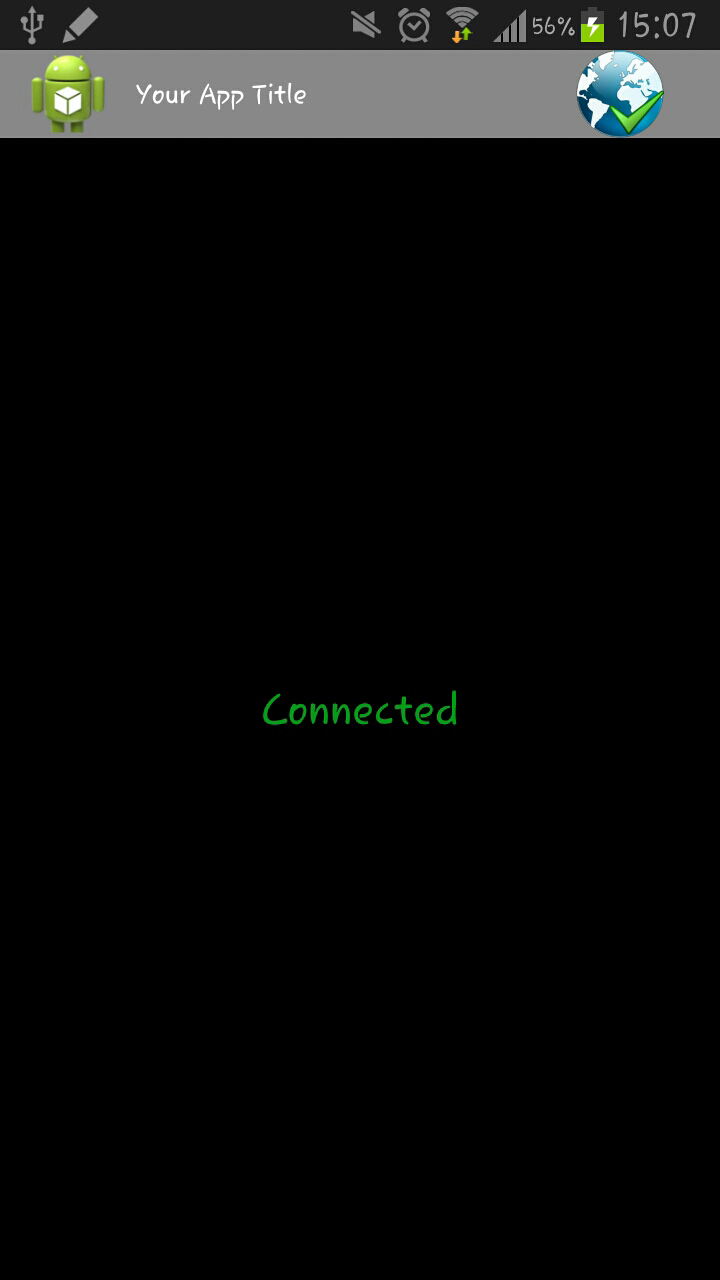
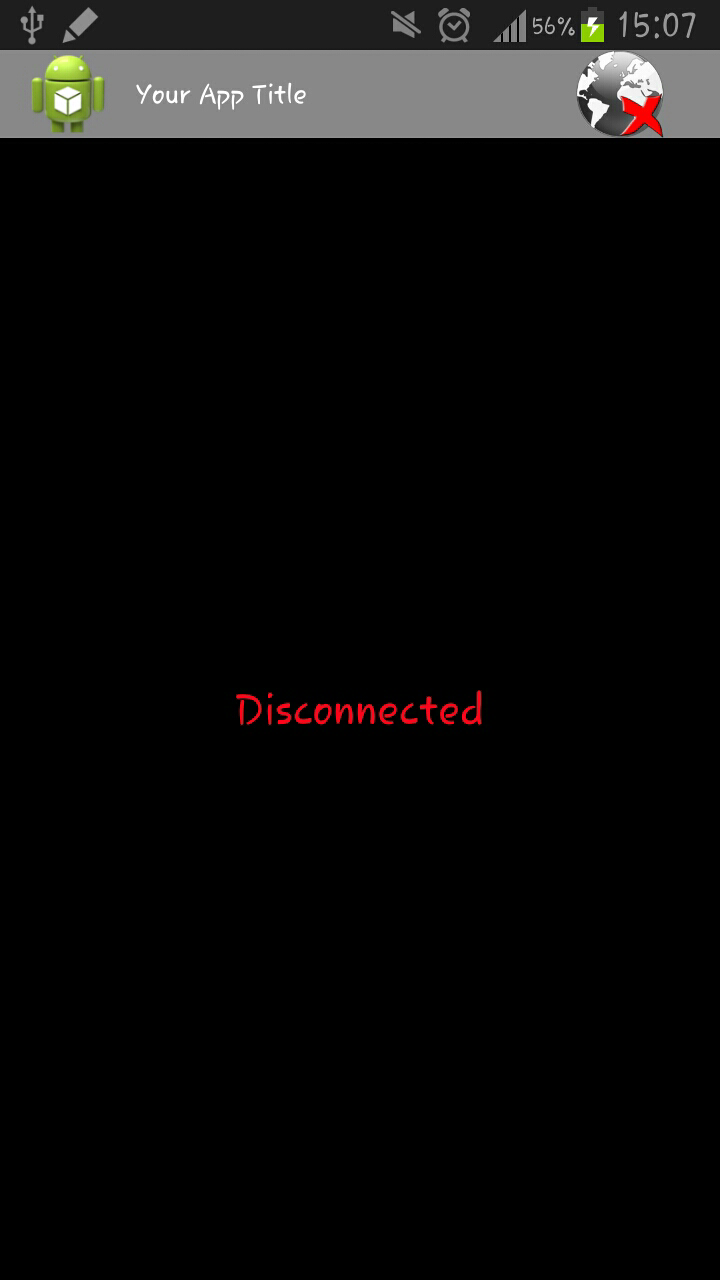
Step 1: Create a new andy project in eclipse. Then in layout folder create a new Android XML file.
Name the file as titlebar.xml
titlebar.xml :
customtheme.xml :
Add ACCESS_NETWORK_STATE in uses permission in AndroidManifest file.
AndroidManifest.xml:
Step 5: Paste the following code in your MainActivity.java class file.
MainActivity.java
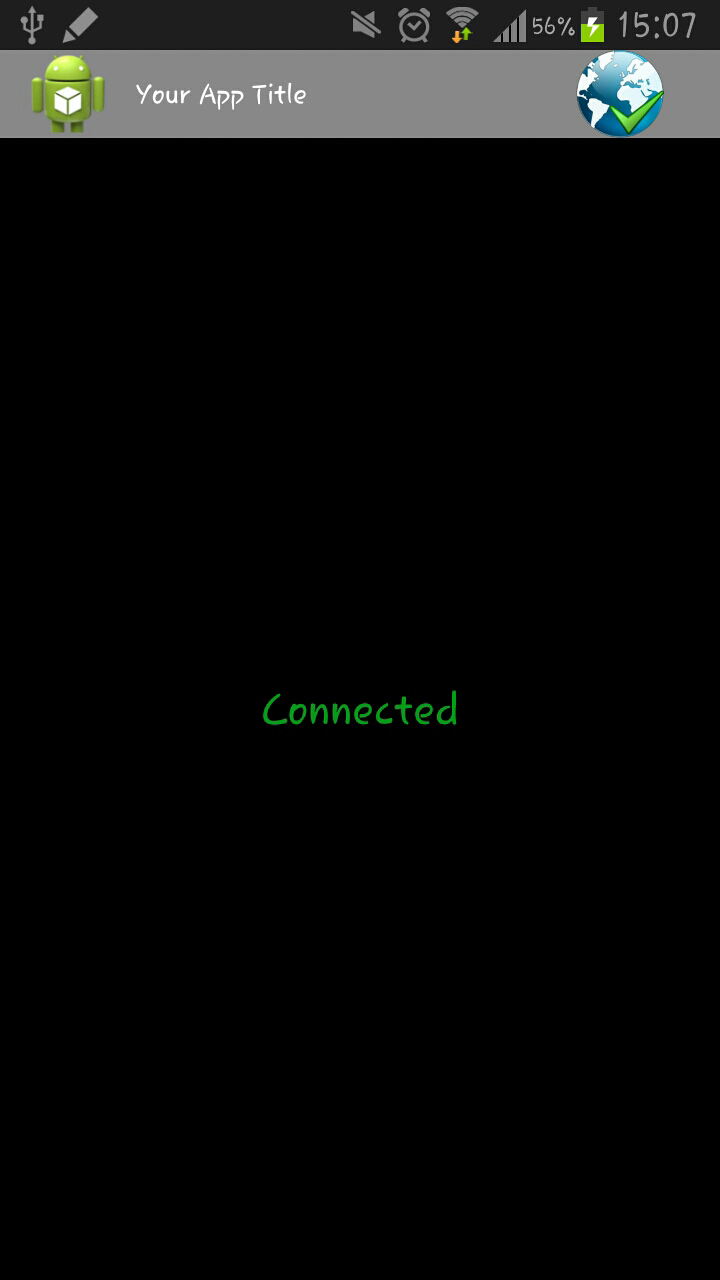
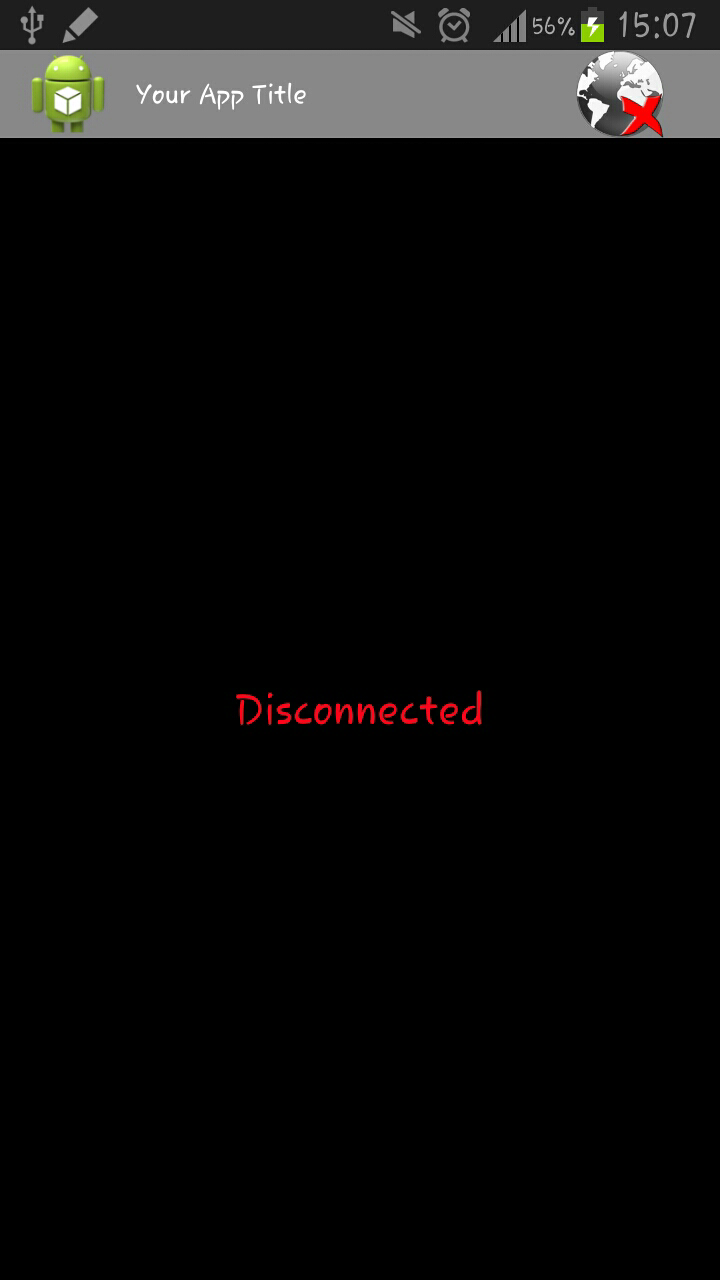
Step 1: Create a new andy project in eclipse. Then in layout folder create a new Android XML file.
Name the file as titlebar.xml
titlebar.xml :
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="44dip"
android:background="#888888"
android:gravity="center_vertical"
android:orientation="horizontal" >
<ImageView
android:id="@+id/appicon"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="left"
android:contentDescription="@string/contentdesc"
android:src="@drawable/ic_launcher"
android:layout_marginLeft="10dp" />
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_toRightOf="@+id/appicon"
android:layout_marginLeft="10dp"
android:text="@string/apptitle"
android:textAppearance="?android:attr/textAppearanceSmall" />
<ImageView
android:id="@+id/netstatimg"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_alignParentTop="true"
android:contentDescription="@string/contentdesc1"
android:gravity="right"
android:src="@drawable/ic_launcher" />
</RelativeLayout>
Step 2: Now create another XML file in res/values Name it as customtheme.xmlcustomtheme.xml :
<?xml version="1.0" encoding="utf-8"?>
<resources>
<style name="appTheme" parent="@android:style/Theme.Black">
<item name="android:textColor">#ffffff</item>
<item name="android:windowTitleBackgroundStyle">@style/titleBarBackground </item>
<item name="android:windowTitleSize">44dip</item>
</style>
<style name="titleBarHeading" parent="@android:style/TextAppearance">
<item name="android:textSize">25sp</item>
<item name="android:textStyle">bold</item>
<item name="android:textColor">#444444</item>
</style>
<style name="titleBarBackground">
<item name="android:background">@android:color/transparent</item>
<item name="android:padding">0px</item>
</style>
</resources>
Step 3: And your Project Manifest file should contain the following code:Add ACCESS_NETWORK_STATE in uses permission in AndroidManifest file.
AndroidManifest.xml:
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/appTheme" >
Step 4: Now paste two images for network connected and disconnected in drawable folder. For good result let the size of the image be 150 x 150 in .png format. Make sure that your images indicates network connectivity and disconnection.Step 5: Paste the following code in your MainActivity.java class file.
MainActivity.java
package com.examp.one;
import android.app.Activity;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.graphics.Color;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.Bundle;
import android.view.Menu;
import android.view.Window;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
private boolean isConnected = false;
TextView st;
private NetworkChangeReceiver receiver;
ImageView imageview;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_CUSTOM_TITLE);
setContentView(R.layout.activity_main);
getWindow().setFeatureInt(Window.FEATURE_CUSTOM_TITLE, R.layout.titlebar);
IntentFilter filter = new IntentFilter(ConnectivityManager.CONNECTIVITY_ACTION);
receiver = new NetworkChangeReceiver();
registerReceiver(receiver, filter);
st=(TextView)findViewById(R.id.status);
imageview = (ImageView)findViewById(R.id.netstatimg);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
public class NetworkChangeReceiver extends BroadcastReceiver {
@Override
public void onReceive(final Context context, final Intent intent) {
System.out.println("Receieved notification about network status");
isNetworkAvailable(context);
}
private boolean isNetworkAvailable(Context context) {
ConnectivityManager connectivity = (ConnectivityManager)
context.getSystemService(Context.CONNECTIVITY_SERVICE);
if (connectivity != null) {
NetworkInfo[] info = connectivity.getAllNetworkInfo();
if (info != null) {
for (int i = 0; i < info.length; i++) {
if (info[i].getState() == NetworkInfo.State.CONNECTED) {
if(!isConnected){
System.out.println("Now you are connected to Internet!"); //prints on logcat
st.setText("Connected");//Set text when connected
st.setTextColor(Color.parseColor("#059e1e"));// set color of text to green
imageview.setImageResource(R.drawable.connected1);//set connected image
Toast.makeText(context, "Connected to Network",
Toast.LENGTH_LONG).show();//make a tost to display connection
isConnected = true;
}
return true;
}}}}
System.out.println("You are not connected to Internet!");//prints on logcat
st.setTextColor(Color.parseColor("#fa051c"));// set color of text to red
st.setText("Disconnected");//Set text when connected
imageview.setImageResource(R.drawable.disconnected1);//set disconnected image
Toast.makeText(context, "Not Connected to any Network",
Toast.LENGTH_LONG).show();// Make a toast to display disconnection
isConnected = false;
return false;
}
}
it works fine..thank u
ReplyDeleteMy pleasure :)
Delete