To set custom font for spinner we need to create our own SpinnerAdapter with our Typeface for getView and getDropDownView().
You will get your font style for spinner in just three simple steps.
To make other customization to your spinner check this link
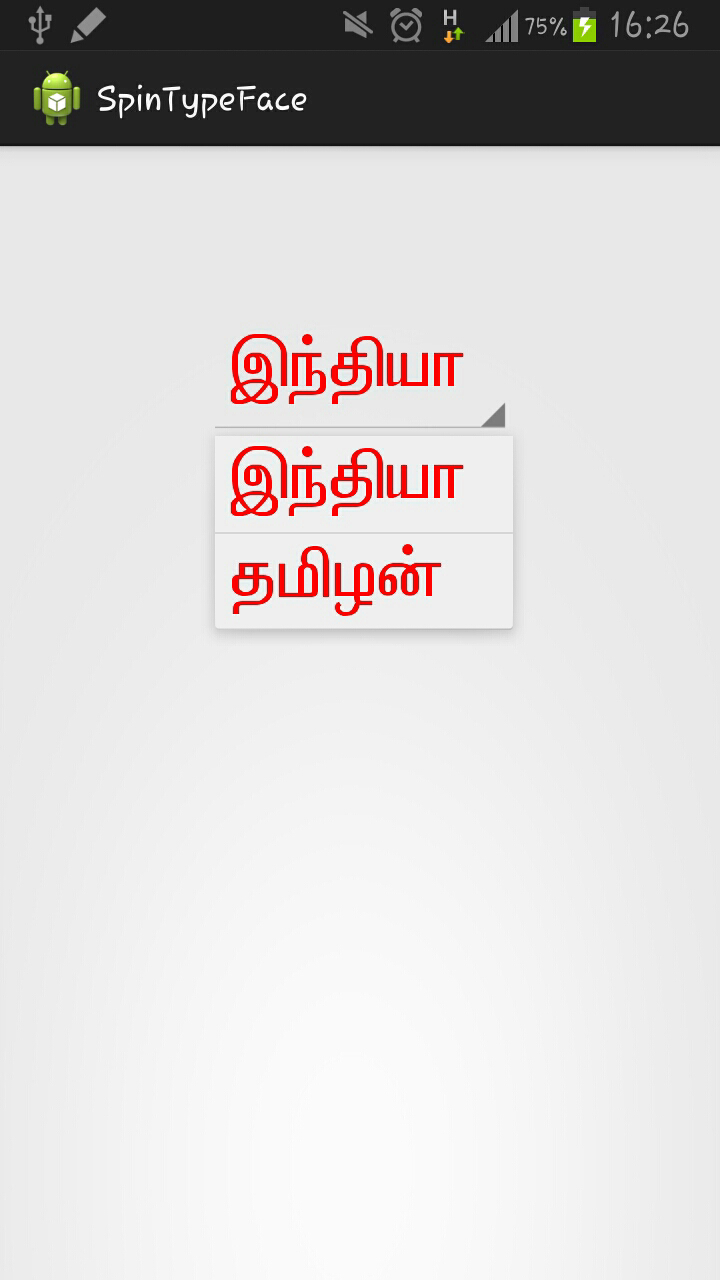
Step 1: Just create a spinner in your layout
activity_main.xml:
Step 3: create a ViewGroup spinner method and call the method.
MainActivity.java:
You will get your font style for spinner in just three simple steps.
To make other customization to your spinner check this link
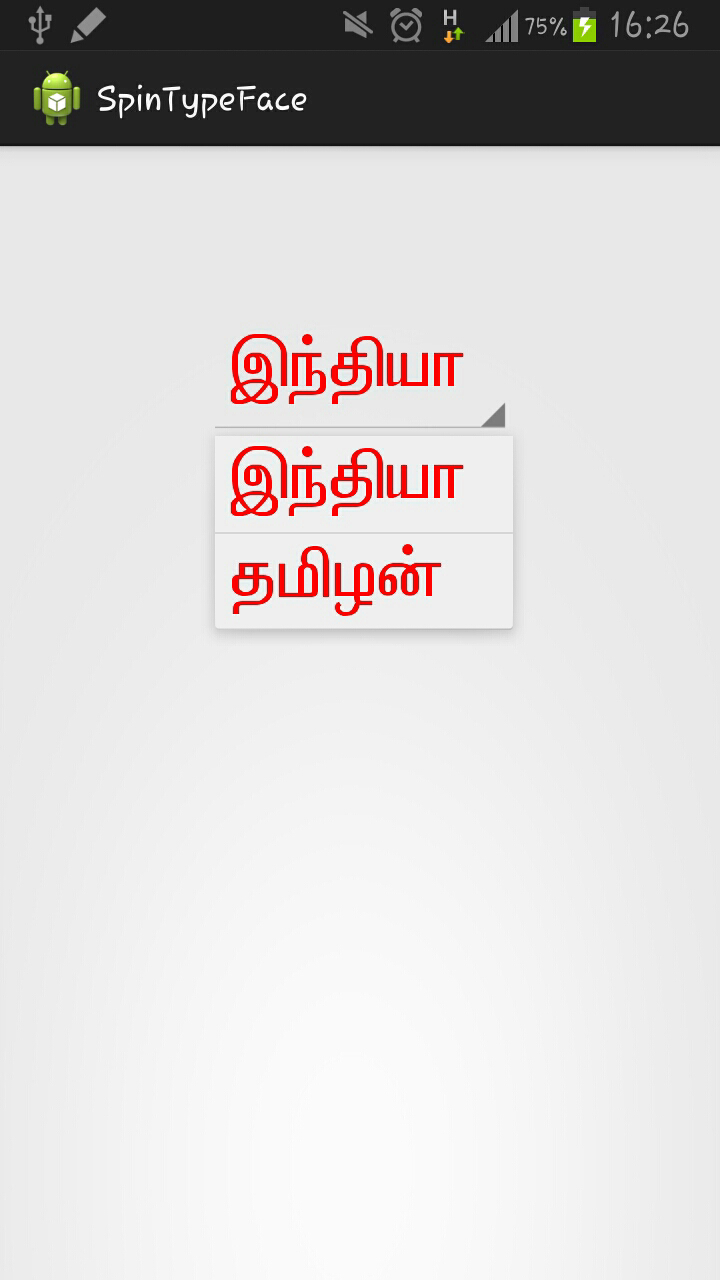
Step 1: Just create a spinner in your layout
activity_main.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<Spinner
android:id="@+id/spinner1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="20dp" />
</RelativeLayout>
Step 2: Create a folder in Assets and name it as fonts. Then paste your typeface (fontname.ttf) file in fonts folder.Step 3: create a ViewGroup spinner method and call the method.
MainActivity.java:
package com.exam.spintypeface;
import android.app.Activity;
import android.graphics.Color;
import android.graphics.Typeface;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.Spinner;
import android.widget.TextView;
public class MainActivity extends Activity {
String list[]={"ϪÂah","jäH‹"};
Typeface tfavv;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
spinner2meth();
}
public <ViewGroup> void spinner2meth(){
Spinner mySpinner = (Spinner) findViewById(R.id.spinner1);
ArrayAdapter<String> adapter1 = new ArrayAdapter<String>(this,android.R.layout.simple_list_item_1, list)
{
public View getView(int position, View convertView, android.view.ViewGroup parent) {
tfavv = Typeface.createFromAsset(getAssets(),"fonts/Avvaiyar.ttf");
TextView v = (TextView) super.getView(position, convertView, parent);
v.setTypeface(tfavv);
v.setTextColor(Color.RED);
v.setTextSize(35);
return v;
}
public View getDropDownView(int position, View convertView, android.view.ViewGroup parent) {
TextView v = (TextView) super.getView(position, convertView, parent);
v.setTypeface(tfavv);
v.setTextColor(Color.RED);
v.setTextSize(35);
return v;
}
};
adapter1.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
mySpinner.setAdapter(adapter1);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}